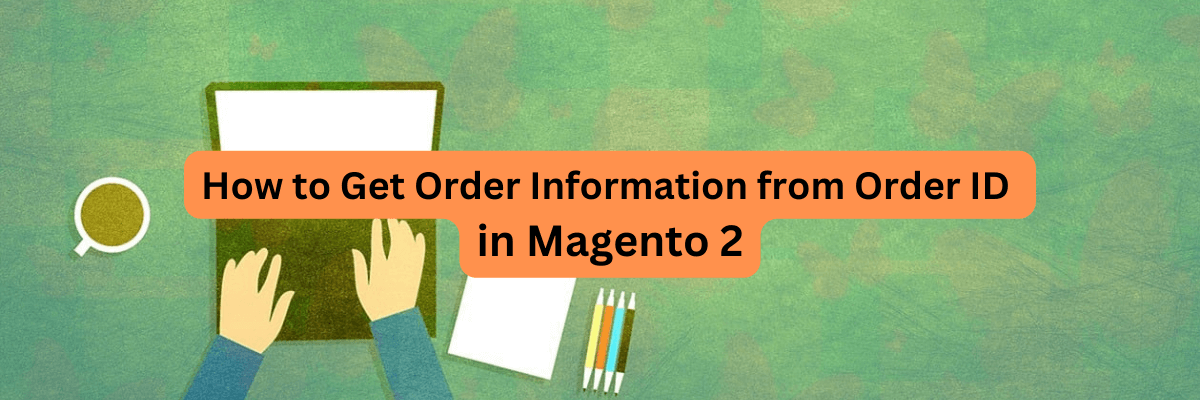
In Magento 2, fetching detailed order information using an order ID is a common task that can be essential for custom modules, reports, and integrations. This blog post will guide you through the process of retrieving comprehensive order data, including order items, payment details, customer information, and billing and shipping addresses. We will explore two main approaches: using the Magento 2 Repository and using the Object Manager.
Using the Repository to Fetch Order Information
Magento 2 encourages using Dependency Injection and Repositories for accessing data rather than directly interacting with the Object Manager. This method adheres to Magento’s best practices and promotes better code maintainability and testing. Below is a detailed example of how to fetch order information using the `OrderRepositoryInterface`
.
Code Snippet:
<?php
namespace Vendor\Module\Class;
class OrderFetcher
{
protected $orderRepository;
public function __construct(
\Magento\Sales\Api\OrderRepositoryInterface $orderRepository
) {
$this->orderRepository = $orderRepository;
}
public function getOrderInformation($orderId)
{
$order = $this->orderRepository->get($orderId);
// Basic Order Information
echo $order->getIncrementId();
echo $order->getGrandTotal();
echo $order->getSubtotal();
// Fetch whole payment information
print_r($order->getPayment()->getData());
// Fetch customer information
echo $order->getCustomerId();
echo $order->getCustomerEmail();
echo $order->getCustomerFirstname();
echo $order->getCustomerLastname();
// Fetch whole billing information
print_r($order->getBillingAddress()->getData());
// Fetch specific billing information
echo $order->getBillingAddress()->getCity();
echo $order->getBillingAddress()->getRegionId();
echo $order->getBillingAddress()->getCountryId();
// Fetch whole shipping information
print_r($order->getShippingAddress()->getData());
// Fetch specific shipping information
echo $order->getShippingAddress()->getCity();
echo $order->getShippingAddress()->getRegionId();
echo $order->getShippingAddress()->getCountryId();
}
}
Explanation
- Dependency Injection: We inject the
OrderRepositoryInterface
into our class, allowing us to access order data in a Magento-compliant manner. - Fetching Order: Using
$this->orderRepository->get($orderId)
, we retrieve the order object. - Order Details: Access various details of the order such as increment ID, grand total, subtotal, payment, customer info, billing address, and shipping address.
- Data Printing: Use
print_r()
to output arrays of data for more comprehensive details.
Using Object Manager to Fetch Order Information
Although using the Object Manager directly is not recommended due to its impact on code maintainability and testing, it can be useful for quick scripts or debugging. Here's how you can retrieve order information using the Object Manager.
Code Snippet:
<?php
$orderId = 2;
$objectManager = \Magento\Framework\App\ObjectManager::getInstance();
$order = $objectManager->create('Magento\Sales\Api\Data\OrderInterface')->load($orderId);
// Fetch whole order information
print_r($order->getData());
// Fetch specific information
echo $order->getIncrementId();
echo $order->getGrandTotal();
echo $order->getSubtotal();
?>
Explanation
- Object Manager Instance: We get an instance of the Object Manager.
- Order Retrieval: Use
$objectManager->create('Magento\Sales\Api\Data\OrderInterface')->load($orderId)
to fetch the order. - Order Details: Output entire order data or specific details as needed.
Fetching Order Items Information
Order items can also be retrieved and examined. Here’s how you can loop through each item in an order to get detailed information.
Code Snippet:
<?php
$orderId = 2;
$objectManager = \Magento\Framework\App\ObjectManager::getInstance();
$order = $objectManager->create('Magento\Sales\Api\Data\OrderInterface')->load($orderId);
// Loop through each item and fetch data
foreach ($order->getAllItems() as $item) {
// Fetch whole item information
print_r($item->getData());
// Fetch specific item information
echo $item->getId();
echo $item->getProductType();
echo $item->getQtyOrdered();
echo $item->getPrice();
}
?>
Explanation
- Retrieve Order: Similar to previous examples, retrieve the order using Object Manager.
- Iterate Items: Use
getAllItems()
to get an array of order items. - Item Details: Output detailed information for each item or specific attributes like ID, product type, ordered quantity, and price.
Conclusion
Fetching order information from an order ID in Magento 2 can be efficiently achieved using either the Repository or Object Manager. While the Repository approach is preferred for its adherence to Magento’s best practices, the Object Manager can be handy for quick operations. Remember to consider the context and requirements of your project to choose the most appropriate method. For production code, always opt for Dependency Injection and avoid direct Object Manager usage to ensure maintainable and testable code.